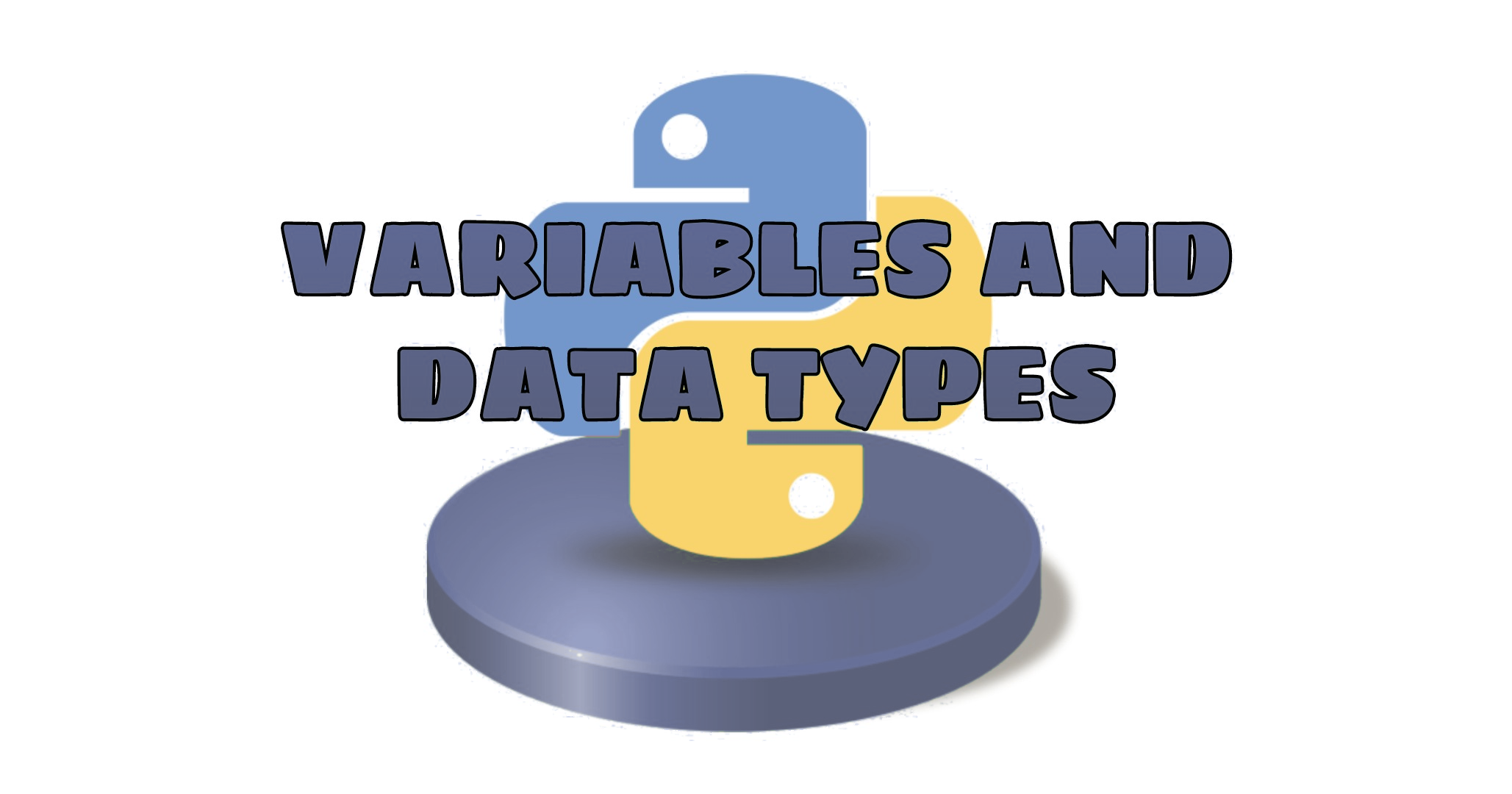
In this article we are going to look at variables in python. We are going to understand variables by answering some basic questions such as, what are variables?, why do we use variables?, How to declare variables?, what are naming conventions for variables?, etc. We are also going to briefly discuss some basic data types such as numbers and strings. We will be understanding every data type in detail in further articles, So let's get started!!!
What are variables and why do we use them?
Variables in python are reserved memory locations. They act as containers to store data. So basically each variable is pointing to a memory location and the value stored in the variable can be accessed by calling the name of the variable.
Suppose you have to write 1000s of lines of code and there is one value which is occuring repeatedly in your code, now suddenly you want to change that value, one thing you can do is go to each line and change that value manually, which is not feasible. Here variables come into picture. You declare a variable once and you store that value in the variable. Now whenever you want that value anywhere in your code you can get it easily by calling the name of the variable, in which that value has been stored. Now suppose you want to change that value, wherever it appears in your code, you can do that easily by changing the value stored in the variable.
How to declare variables in python?
There is no specific command to declare variables in python. Declaring a variable is pretty straightforward and easy. You just write the name of the variable and assign a value to it.
As we discussed earlier the value stored in a variable can be easily accessed by calling the name of that variable so now, let's print the values we stored in our variables. We can easily do that by using the print
statement in python. The print statement is a built-in function in python which we will be discussing in detail when we look at built-in functions.
To print the value stored in a variable just pass the name of that variable as a parameter into the print function print(variable_name)
.
Variable assignment
Assigning a value to a variable
As we discussed earlier, there is no special command to assign a value to a variable. To declare a variable just write the name of the variable and assign a value to it. If you change the value of an existing variable i.e. if you assign a new value to a pre-existing variable, the old value will be discarded.
Assigning the value of one variable to another
You can assign the value of one variable to another.
Assigning one value to multiple variables
Python allows chained assignment, which makes it possible to assign the same value to multiple variables simultaneously.
Assigning many values to multiple variables
You can assign many values to multiple variables simultaneously.
Variable naming conventions
There are certain rules you need to follow while naming variables in python.
- Rule-1: You should start variable name with an alphabet or underscore(_) character.
- Rule-2: A variable name can only contain A-Z, a-z, 0-9 and underscore(_).
- Rule-3: You cannot start the variable name with a number.
- Rule-4: You cannot use special characters with the variable name such as such as $,%,#,&,@.-,^ etc.
- Rule-5: Variable names are case sensitive. For example
var
andVar
are two different variables. - Rule-6: Do not use reserved keywords as a variable names.
Reserved keywords
There are certain keywords in python which are reserved to perform specific operations. You are not supposed to use these keywords as variable names.
and |
as |
assert |
break |
class |
continue |
def |
del |
elif |
else |
except |
false |
finally |
for |
from |
global |
if |
import |
is |
in |
lambda |
None |
nonlocal |
not |
or |
pass |
raise |
return |
True |
try |
while |
with |
yield |
Object Referencing and Identity
What exactly happens when we assign a value to a variable in python? The python variable is a reserved memory location i.e. when you declare a variable, that variable is pointing to the memory location, where the assigned value has been stored. So basically a variable is a name given to the memory location where the object
is stored and you can get the object by calling the name of that variable.
Python is a an object-oriented language so basically every item of data (variables) in Python is an object of a specific type or class. We will be discussing this in detail when we look at object-oriented programming
in python.
Variable types
Data types in python are a way or categorizing or classifying data into different types. A data type tells us what kind of operations can be performed on that particular data. There are 7 different data types in python Numbers
, strings
, Lists
, Tuples
, Sets
, Dictionaries
and Booleans
. We will discussing all these data types in detail later.
Variables in python can belong to different data types based on the data assigned to them.
As we can see above the first variable var1
belongs to integer type and var2
belongs float type and both these integer and float types come under the data type number
. Similarly the variable var3
belongs to string
data type.
Casting
In python there is no need of specifying the data type of an object while declaring a variable. Python dynamically allocates the data type based on the value assigned to the variable.
If you want to specify the data type of a variable, it can be done by casting.
Here we are using built-in functions int()
(integer), float()
(float) and str()
(string) to specify the data type for variables
Object id
We know that variables are containers to store data and they point to a memory location. If two or more variables have the same value then they point to the same memory location. Let's suppose we have three variables var1
, var2
and var3
, all have the same value 20, then all these variables will be pointing to the same memory location
Now if we change the value of var2
, then var2
will be pointing to a new memory location.
We can verify the above statements by using the id()
function. The id() function is a built-in function in python and it gives us a unique id for a variable. This unique id represents the memory location where the variable is pointing. We will revisit the id() function when we will be looking at built-in functions in python.
Conclusion
In this article, we looked at variables in python. We understood what are variables, how to declare variables and the different methods of assigning a value to a variable. We also understood memory referencing and object identity of variables by using built-in functions in python. We also looked at naming conventions for variables and discussed the python code for every section.
You can download the jupyter notebook for above post here